简介
render-markdown-with-position
是一个专门用于在前端将 markdown 文本渲染成 HTML,并且对于每个渲染的元素会保存它在原文的位置,方便进行跳转、定位等操作的 npm 包。
该 npm 包主要适用于在前端展示文档、博客等需要展示 markdown 内容的场景。使用该 npm 包可以帮助我们更好的处理 markdown 内容,并且方便我们进行各种操作。
安装
render-markdown-with-position
可以直接通过 npm 安装,使用如下命令即可:
npm install render-markdown-with-position
使用方法
使用 render-markdown-with-position
的方法非常简单,只需要引入该包之后,调用其 render
方法即可完成简单的 markdown 渲染。
import { render } from 'render-markdown-with-position' const text = '# Hello World' const result = render(text) console.log(result)
该代码将输出一个包含 markdown 渲染结果的 HTML 字符串。
深入了解
除了简单的使用之外,render-markdown-with-position
还支持一些高级的功能,这些功能在实际使用场景中非常有用。
块级元素
在 markdown 中,存在各种各样的块级元素,如标题、段落、列表、表格等等。render-markdown-with-position
支持大多数 markdown 中的块级元素,并且对于每个块级元素都会附带它在原文中的位置信息,方便我们进行一些操作。
标题
渲染 markdown 标题是非常简单的,只需要在文本开头使用 "#" 即可。
import { render } from 'render-markdown-with-position' const text = '# Hello World' const result = render(text) console.log(result)
对于一个标题元素,其位置信息包含了该标题在原文中的开始位置和结束位置,代码示例:
const text = '# Hello World' const result = render(text) console.log(result[0].position) // [0, 12]
段落
渲染 markdown 段落也非常简单,只需要直接书写即可。
import { render } from 'render-markdown-with-position' const text = 'This is a paragraph.' const result = render(text) console.log(result)
对于一个段落元素,其位置信息同样包含了该段落在原文中的开始位置和结束位置,代码示例:
const text = 'This is a paragraph.' const result = render(text) console.log(result[0].position) // [0, 20]
列表
渲染 markdown 列表需要使用特定的标记符,如:"- " 或者 "1. " 等等。render-markdown-with-position
支持多种列表风格,并且对于每个列表项也会保存其在原文中的位置信息。
-- -------------------- ---- ------- ------ - ------ - ---- ------------------------------- ----- ---- - - - ---- - - ---- - - ---- - - ----- ------ - ------------ -------------------
对于一个列表元素,其位置信息包含了该列表在原文中的开始位置和结束位置,以及每个列表项在原文中的位置信息,代码示例:
-- -------------------- ---- ------- ----- ---- - - - ---- - - ---- - - ---- - - ----- ------ - ------------ ------------------------------- -- --- --- ------------------------------------------- -- --- -- ------------------------------------------- -- ---- --- ------------------------------------------- -- ---- ---
这个示例代码包含了一个包含三个列表项的列表,可以看到使用 render
函数渲染的结果中包含了整个列表在原文中的位置信息,以及每个列表项在原文中的位置信息。
表格
渲染 markdown 表格同样需要使用特定的标记符,如:"|" 等等。render-markdown-with-position
支持渲染基本表格,并且对于每个单元格也会保存其在原文中的位置信息。
-- -------------------- ---- ------- ------ - ------ - ---- ------------------------------- ----- ---- - - - ------ - - ------ - - - --- - --- - - --- -- ------ - - --- -- ------ - - - --- -- ------ - - --- -- ------ - - - ----- ------ - ------------ -------------------
对于一个表格元素,其位置信息包含了该表格在原文中的开始位置和结束位置,以及每个单元格在原文中的位置信息,代码示例:
-- -------------------- ---- ------- ----- ---- - - - ------ - - ------ - - - --- - --- - - --- -- ------ - - --- -- ------ - - - --- -- ------ - - --- -- ------ - - - ----- ------ - ------------ ------------------------------- -- --- --- ------------------------------------------- -- --- --- ------------------------------------------- -- ---- --- ------------------------------------------------------- -- ---- --- ------------------------------------------------------- -- ---- --- ------------------------------------------------------- -- ---- --- ------------------------------------------------------- -- ---- ----
这个示例代码包含了一个包含两行、两列的表格,可以看到使用 render
函数渲染的结果中包含了整个表格在原文中的位置信息,以及每个单元格在原文中的位置信息。
内联元素
在 markdown 中,各种内联元素如:加粗、链接、图片等等都是非常常见的。render-markdown-with-position
同样支持大多数的内联元素,并且对于每个内联元素也会保存其在原文中的位置信息。
加粗
加粗可以使用 "**" 或者 "__" 包裹文本,如:**Hello World**
。使用 render-markdown-with-position
渲染 markdown 时,会将加粗的文本转换为纯 HTML。
import { render } from 'render-markdown-with-position' const text = '**Hello World**' const result = render(text) console.log(result)
对于一个加粗元素,其位置信息包含了该加粗元素在原文中的开始位置和结束位置,代码示例:
const text = '**Hello World**' const result = render(text) console.log(result[0].position) // [0, 14]
链接
链接可以使用 "text" 的形式书写,如:[github](https://github.com)
。使用 render-markdown-with-position
渲染 markdown 时,会将链接转换为 HTML <a>
标签。
import { render } from 'render-markdown-with-position' const text = '[github](https://github.com)' const result = render(text) console.log(result)
对于一个链接元素,其位置信息包含了该链接元素在原文中的开始位置和结束位置,代码示例:
const text = '[github](https://github.com)' const result = render(text) console.log(result[0].position) // [0, 22]
图片
图片可以使用 "" 的形式书写,如:
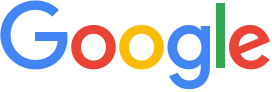
。使用 render-markdown-with-position
渲染 markdown 时,会将图片转换为 HTML <img>
标签。
import { render } from 'render-markdown-with-position' const text = '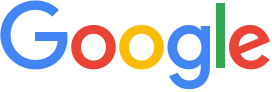' const result = render(text) console.log(result)
对于一个图片元素,其位置信息包含了该图片元素在原文中的开始位置和结束位置,代码示例:
const text = '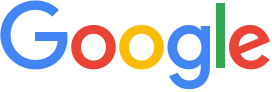' const result = render(text) console.log(result[0].position) // [0, 73]
案例
在实际使用过程中,render-markdown-with-position
可以帮助我们快速、准确地展示和操作 markdown 内容。比如,我们可以基于该 npm 包快速地实现一个 markdown 阅读器,方便我们查看和阅读各种文档和博客。
以下是一个简单的 markdown 阅读器实现代码示例:
-- -------------------- ---- ------- --------- ----- ------ ------ --------------- -------------- ------- ------ ---- ------------------------------ ------- --------------------------------------------------------------------------------------------------------- -------- ----------------- -------------- -- ---------------- ---------- -- - ----- --------- - --------------------------------------------- ------------------- - ---------------------------------------------- ---------------------------------------------------------- -- - ----- -------- - ---------------------------------- ------------------------------ -- -- - ---------- ------- -- -------- ------------- -- -- -- --------- ------- -------
该代码通过 AJAX 加载一个 markdown 文件,并将该文件渲染成 HTML 并显示在页面上。并且,当用户点击某个元素时,弹出该元素在 markdown 文本中的位置信息。通过这个例子可以看到,render-markdown-with-position
可以帮助我们非常方便地展示和操作 markdown 内容。
总结
render-markdown-with-position
是一个非常实用、好用的 npm 包,在前端对于 markdown 渲染和操作方面提供了非常好的支持。通过本文的介绍和实例,相信读者已经对该 npm 包有了更深入的了解,期待各位读者可以将该 npm 包运用到实际项目中,提升自己的开发效率。
来源:JavaScript中文网 ,转载请注明来源 https://www.javascriptcn.com/post/600552d981e8991b448d043f